How to Scrape Google Maps Reviews

In this article, I’ll walk you through how to scrape Google Maps data using three different approaches. Each method has its own pros and cons, so you can pick the one that works best for your skills and needs. The three approaches I’ll be covering are as follows:
- Writing Your Own Python Script. I’ll guide you step by step on how to build a custom scraper on Python. Plus, I’ll share ready-to-use code that you can modify to fit your specific requirements.
- Using a Google Maps Reviews API. If coding isn’t your thing (or you’d rather not reinvent the wheel), this option lets you pull reviews via an API. You’ll still need some programming knowledge, but it’s much simpler than creating a new scraper. In this approach it’s all about handling the data you receive.
- Using a No-Code Google Maps Reviews Scraper. Don’t want to touch code at all? No problem. I’ll introduce you to a ready-made tool that lets you scrape Google reviews without any programming skills. Just point, click, and you’re good to go.
Feel free to jump directly to the method that catches your attention to get all the details.
Method 1: Scrape Google Maps Reviews with Python
Suppose you’re seeking more than just the basic information about locations on Google Maps, for example you also want to extract reviews. In this case, you’ll quickly discover that utilizing basic libraries such as Requests with BeautifulSoup or even Scrapy is insufficient. To build a scraper for this, you’ll need something more functional, like Selenium. It’s a powerful library that allows you to retrieve data from a page and interact with it.
Extract Google Maps Reviews for a Specific Place
We’ll go over each step of this script below, but for those who want TL;DR version and to get straight to the result, here’s the full final script right away:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.options import Options
import time
url = "https://www.google.com/maps/place/Joe's+Pizza+Broadway/@40.7546795,-73.9870291,17z/data=!3m1!5s0x89c259ab3e91ed73:0x4074c4cfa25e210b!4m18!1m9!3m8!1s0x89c259ab3c1ef289:0x3b67a41175949f55!2sJoe's+Pizza+Broadway!8m2!3d40.7546795!4d-73.9870291!9m1!1b1!16s%2Fg%2F11bw4ws2mt!3m7!1s0x89c259ab3c1ef289:0x3b67a41175949f55!8m2!3d40.7546795!4d-73.9870291!9m1!1b1!16s%2Fg%2F11bw4ws2mt?entry=ttu&g_ep=EgoyMDI0MTIxMS4wIKXMDSoASAFQAw%3D%3D"
chrome_options = Options()
driver = webdriver.Chrome(options=chrome_options)
driver.get(url)
time.sleep(5)
reviews = []
review_elements = driver.find_elements(By.CSS_SELECTOR, 'div.jftiEf')
for review_elem in review_elements:
reviewer_name = review_elem.find_element(By.CSS_SELECTOR, '.d4r55').text.strip() if review_elem.find_element(By.CSS_SELECTOR, '.d4r55') else 'No name'
review_text = review_elem.find_element(By.CSS_SELECTOR, '.wiI7pd').text.strip() if review_elem.find_element(By.CSS_SELECTOR, '.wiI7pd') else 'No review text'
rating_elem = review_elem.find_element(By.CSS_SELECTOR, '.hCCjke')
rating = len(rating_elem.find_elements(By.CSS_SELECTOR, '.NhBTye')) if rating_elem else 0
date_elem = review_elem.find_element(By.CSS_SELECTOR, '.rsqaWe')
review_date = date_elem.text.strip() if date_elem else 'No date'
photo_links = []
photo_elements = review_elem.find_elements(By.CSS_SELECTOR, '.Tya61d')
for photo_elem in photo_elements:
photo_url = photo_elem.get_attribute('style')
if photo_url:
start = photo_url.find('url("') + len('url("')
end = photo_url.find('")')
photo_links.append(photo_url[start:end])
reviews.append({
'reviewer_name': reviewer_name,
'review_text': review_text,
'rating': rating,
'review_date': review_date,
'photo_links': photo_links
})
for review in reviews:
print(f"Author: {review['reviewer_name']}")
print(f"Review: {review['review_text']}")
print(f"Rating: {review['rating']} stars")
print(f"Review date: {review['review_date']}")
print("Photo links:")
for link in review['photo_links']:
print(f" {link}")
print("-" * 50)
driver.quit()
In this script, you’ll need to provide the link to the reviews page of the place you want to scrape. Before running it, make sure you’ve updated the URL and double-checked the selectors – Google likes to change class names often. If there have been recent Google updates, things might not run properly.
Let’s break this script down step by step. First, you’ll need to import the required libraries and modules:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.options import Options
import time
Next, we set the URL for the place and initialize the Selenium Chrome driver:
url = "https://www.google.com/maps/place/Joe's+Pizza+Broadway/@40.7546795,-73.9870291,17z/data=!3m1!5s0x89c259ab3e91ed73:0x4074c4cfa25e210b!4m18!1m9!3m8!1s0x89c259ab3c1ef289:0x3b67a41175949f55!2sJoe's+Pizza+Broadway!8m2!3d40.7546795!4d-73.9870291!9m1!1b1!16s%2Fg%2F11bw4ws2mt!3m7!1s0x89c259ab3c1ef289:0x3b67a41175949f55!8m2!3d40.7546795!4d-73.9870291!9m1!1b1!16s%2Fg%2F11bw4ws2mt?entry=ttu&g_ep=EgoyMDI0MTIxMS4wIKXMDSoASAFQAw%3D%3D"
chrome_options = Options()
driver = webdriver.Chrome(options=chrome_options)
Navigate to the page and add a small delay to give it time to load:
driver.get(url)
time.sleep(5)
Now comes the detective work – analyzing the page to find out the correct selectors for the elements you want to scrape:
Data | CSS Selector |
---|---|
Reviewer Name | div.jftiEf .d4r55 |
Review Text | div.jftiEf .wiI7pd |
Rating | div.jftiEf .hCCjke .NhBTye |
Review Date | div.jftiEf .rsqaWe |
Photo Links | div.jftiEf .Tya61d |
Unfortunately, the page doesn’t always have clear and simple selectors. To make things more complicated, the class names often change frequently. You’ll need to verify them before running the script to ensure they’re still valid.
Once you have the selectors, we can return to the script and collect the data:
reviews = []
review_elements = driver.find_elements(By.CSS_SELECTOR, 'div.jftiEf')
for review_elem in review_elements:
reviewer_name = review_elem.find_element(By.CSS_SELECTOR, '.d4r55').text.strip() if review_elem.find_element(By.CSS_SELECTOR, '.d4r55') else 'No name'
review_text = review_elem.find_element(By.CSS_SELECTOR, '.wiI7pd').text.strip() if review_elem.find_element(By.CSS_SELECTOR, '.wiI7pd') else 'No review text'
rating_elem = review_elem.find_element(By.CSS_SELECTOR, '.hCCjke')
rating = len(rating_elem.find_elements(By.CSS_SELECTOR, '.NhBTye')) if rating_elem else 0
date_elem = review_elem.find_element(By.CSS_SELECTOR, '.rsqaWe')
review_date = date_elem.text.strip() if date_elem else 'No date'
photo_links = []
photo_elements = review_elem.find_elements(By.CSS_SELECTOR, '.Tya61d')
for photo_elem in photo_elements:
photo_url = photo_elem.get_attribute('style')
if photo_url:
start = photo_url.find('url("') + len('url("')
end = photo_url.find('")')
photo_links.append(photo_url[start:end])
reviews.append({
'reviewer_name': reviewer_name,
'review_text': review_text,
'rating': rating,
'review_date': review_date,
'photo_links': photo_links
})
Finally, we print the data and close the webdriver.
for review in reviews:
print(f"Author: {review['reviewer_name']}")
print(f"Review: {review['review_text']}")
print(f"Rating: {review['rating']} stars")
print(f"Review date: {review['review_date']}")
print("Photo links:")
for link in review['photo_links']:
print(f" {link}")
print("-" * 75)
driver.quit()
Here’s what you’ll get as a result:
D:\scripts\google maps reviews python>reviews_place.py
DevTools listening on ws://127.0.0.1:49559/devtools/browser/494e-9fe7-57b3f0ef1fdd
Author: deinz abella
Review: Visited twice in this location, there was a line both times but the wait wasn’t bad at all. Moves fast. I got the cheese pizza, I guess it’s the classic must try, that was good. I came back for the got their version of supreme pizza with …
Rating: 0 stars
Review date: a week ago
Photo links:
https://lh5.googleusercontent.com/p/AF1QipP7ZIsdTKiFrN1hORnKIR6q0A9wJNPdn6ID72Xp=w375-h281-p-k-no
https://lh5.googleusercontent.com/p/AF1QipPTdVki6E1lkviWA9LeaUjGeKmi6S_-paRkaklc=w375-h281-p-k-no
https://lh5.googleusercontent.com/p/AF1QipO_C3OqQqspqiucL7sVNZJPaoa65GgjZzPzoI9_=w375-h281-p-k-no
https://lh5.googleusercontent.com/p/AF1QipP6nbHmt3lcezjCAgPxR2C8KLZvYVtATd67m7aX=w375-h281-p-k-no
---------------------------------------------------------------------------------
Author: Megan
Review: Joe's Pizza is absolutely delicious—easily the best pizza I’ve ever had! Now I completely understand what all the NYC pizza hype is about. The crust is perfectly crispy, the sauce is rich and flavorful, and the cheese is just the right …
Rating: 0 stars
Review date: 4 days ago
Photo links:
https://lh5.googleusercontent.com/p/AF1QipNEGSasQOx6peXD9ruYnmNq6dD9MYAcX8sxBLzR=w375-h563-p-k-no
https://lh5.googleusercontent.com/p/AF1QipOIDq9dhO2gPmNlAhlRErwA5hAnvu-r1doRvZYM=w375-h563-p-k-no
---------------------------------------------------------------------------------
Author: Kimberly Hope 1111
Review: You can tell everything you need to know by the simple and classic cheese pizza, which is what we opted for...Very delicious! …
Rating: 0 stars
Review date: a week ago
Photo links:
https://lh5.googleusercontent.com/p/AF1QipMb-dOCSdXLovTdlwx1CO_1YLDycM_uW8t8T_DE=w375-h281-p-k-no
https://lh5.googleusercontent.com/p/AF1QipOVNSqraRl5aKeosYFgGan_GqpsgoQ930_gKiDk=w375-h281-p-k-no
https://lh5.googleusercontent.com/p/AF1QipN-I_49uFDUc18nJMWnC55bqCZ_5RakREhFEPA2=w375-h281-p-k-no
https://lh5.googleusercontent.com/p/AF1QipMzXTPIz2Xk0Q-kHX8-KcwwLurXREDAZA4rOoRC=w375-h281-p-k-no
This script will scrape the first eleven reviews from the page. To load reviews from other pages, you’ll need to implement infinite scrolling to load additional reviews from Google Maps.
Scrape Google Reviews for Multiple Places
We need to modify the script we discussed earlier to find places based on a specific query and gather customer feedback from all relevant locations. If you want only the code, here’s the final version:
import json
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.options import Options
import time
keyword = "pizza in new york usa places" # Change keyword here
chrome_options = Options()
driver = webdriver.Chrome(options=chrome_options)
def collect_place_links(keyword):
driver.get(f"https://www.google.com/maps/search/{keyword.replace(' ', '+')}/")
time.sleep(3)
return [elem.get_attribute('href') for elem in driver.find_elements(By.CSS_SELECTOR, 'a.hfpxzc')]
def scrape_reviews():
reviews = []
for review_elem in driver.find_elements(By.CSS_SELECTOR, 'div.jftiEf'):
reviewer_name = review_elem.find_element(By.CSS_SELECTOR, '.d4r55').text.strip() if review_elem.find_elements(By.CSS_SELECTOR, '.d4r55') else 'No name'
review_text = review_elem.find_element(By.CSS_SELECTOR, '.wiI7pd').text.strip() if review_elem.find_elements(By.CSS_SELECTOR, '.wiI7pd') else 'No review text'
rating = len(review_elem.find_elements(By.CSS_SELECTOR, '.NhBTye')) if review_elem.find_elements(By.CSS_SELECTOR, '.hCCjke') else 0
review_date = review_elem.find_element(By.CSS_SELECTOR, '.rsqaWe').text.strip() if review_elem.find_elements(By.CSS_SELECTOR, '.rsqaWe') else 'No date'
photo_links = [photo.get_attribute('style').split('url("')[1].split('")')[0] for photo in review_elem.find_elements(By.CSS_SELECTOR, '.Tya61d')]
reviews.append({
'reviewer_name': reviewer_name,
'review_text': review_text,
'rating': rating,
'review_date': review_date,
'photo_links': photo_links
})
return reviews
def navigate_to_reviews(links):
all_reviews = {}
for href in links:
driver.get(href)
time.sleep(3)
try:
driver.find_element(By.XPATH, "//button[contains(@aria-label, 'Reviews')]").click()
time.sleep(3)
all_reviews[href] = scrape_reviews()
print(f"Scraped reviews for {href}")
except Exception as e:
print(f"Failed to extract reviews for {href}: {str(e)}")
return all_reviews
def save_to_file(data, filename="reviews.json"):
with open(filename, 'w', encoding='utf-8') as f:
json.dump(data, f, ensure_ascii=False, indent=4)
print(f"Data saved to {filename}")
links = collect_place_links(keyword)
all_reviews = navigate_to_reviews(links)
save_to_file(all_reviews)
driver.quit()
In this version, we’ve added functionality to search for places using a keyword, collect links to multiple locations, and extract reviews for each location. We save the data in a JSON file because printing everything to the console would be overwhelming. After running the script, we end up with 204 reviews from different places.
The review-scraping part from the previous example has been refactored into a separate function, scrape_reviews(), so we won’t repeat that here. To collect links to different locations on Google Maps, we generate a search URL using a keyword from a variable and then extract all the links matching a specific selector:
def collect_place_links(keyword):
driver.get(f"https://www.google.com/maps/search/{keyword.replace(' ', '+')}/")
time.sleep(3)
return [elem.get_attribute('href') for elem in driver.find_elements(By.CSS_SELECTOR, 'a.hfpxzc')]
Next, we loop through the collected links, navigate to the “Reviews” section on each page, and scrape the reviews:
def navigate_to_reviews(links):
all_reviews = {}
for href in links:
driver.get(href)
time.sleep(3)
try:
driver.find_element(By.XPATH, "//button[contains(@aria-label, 'Reviews')]").click()
time.sleep(3)
all_reviews[href] = scrape_reviews()
print(f"Scraped reviews for {href}")
except Exception as e:
print(f"Failed to scrape Google reviews for {href}: {str(e)}")
return all_reviews
Finally, we save all the data to a file:
def save_to_file(data, filename="reviews.json"):
with open(filename, 'w', encoding='utf-8') as f:
json.dump(data, f, ensure_ascii=False, indent=4)
print(f"Data saved to {filename}")
Here’s an example of what the JSON output looks like:
{
"https://www.google.com/maps/place/Joe%27s+Pizza+Broadway/data=!4m7!3m6!1s0x89c259ab3c1ef289:0x3b67a41175949f55!8m2!3d40.7546795!4d-73.9870291!16s%2Fg%2F11bw4ws2mt!19sChIJifIePKtZwokRVZ-UdRGkZzs?authuser=0&hl=en&rclk=1": [
{
"reviewer_name": "deinz abella",
"review_text": "Visited twice in this location, there was a line both times but the wait wasn’t bad at all. Moves fast. I got the cheese pizza, I guess it’s the classic must try, that was good. I came back for the got their version of supreme pizza with …",
"rating": 5,
"review_date": "a week ago",
"photo_links": [
"https://lh5.googleusercontent.com/p/AF1QipP7ZIsdTKiFrN1hORnKIR6q0A9wJNPdn6ID72Xp=w375-h281-p-k-no",
"https://lh5.googleusercontent.com/p/AF1QipPTdVki6E1lkviWA9LeaUjGeKmi6S_-paRkaklc=w375-h281-p-k-no",
"https://lh5.googleusercontent.com/p/AF1QipO_C3OqQqspqiucL7sVNZJPaoa65GgjZzPzoI9_=w375-h281-p-k-no",
"https://lh5.googleusercontent.com/p/AF1QipP6nbHmt3lcezjCAgPxR2C8KLZvYVtATd67m7aX=w375-h281-p-k-no"
]
},
…
}
If using Selenium for web scraping seems like a hassle, don’t worry – we’ll explore a much simpler and faster way to gather Google reviews next.
Method 2: Scrape Google Maps Reviews Using an API
The second method is simpler because it uses a ready-made API to fetch all the required data based on specified filters. All we have to do is send a request to the Google Maps Reviews API, retrieve the data, and save it in the format we want.
To run the code examples from this section, you’ll need to provide your personal HasData API key. You can get one for free by signing up on our website.
Scrape Reviews by PlaceID&DataID
If you want to extract reviews for a specific place, this example is exactly what you need. Let me walk you through it step by step. First, we’ll start with the full code and then break it down below:
import requests
import csv
API_KEY = 'YOUR-API-KEY'
identifier = "dataId" # Change to "placeId" if needed
identifier_value = "0x80cc0654bd27e08d%3A0xb1c2554442d42e8d"
url = f"https://api.hasdata.com/scrape/google-maps/reviews?{identifier}={identifier_value}"
headers = {
'Content-Type': 'application/json',
'x-api-key': API_KEY
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
data = response.json()
with open('reviews.csv', mode='w', newline='', encoding='utf-8') as file:
writer = csv.writer(file)
writer.writerow(['User Name', 'User Link', 'Rating', 'Date', 'Snippet', 'Review Link', 'Likes'])
for review in data.get('reviews', []):
writer.writerow([
review['user'].get('name', 'N/A'),
review['user'].get('link', 'N/A'),
review.get('rating', 'N/A'),
review.get('date', 'N/A'),
review.get('snippet', 'N/A'),
review.get('link', 'N/A'),
review.get('likes', 'N/A')
])
else:
print(f"Error. Status Code: {response.status_code}")
Now, let’s break it down so you can see how everything fits together. We’ll start by importing the necessary libraries to handle API requests and save the data into a file:
import requests
import csv
Next, we’ll create variables to store HasData’s API key and the type of identifier we’re working with. The identifier could be either a placeId or a dataId, depending on how the location is defined:
API_KEY = 'YOUR-API-KEY'
identifier = "dataId" # Change to "placeId" if needed
identifier_value = "0x80cc0654bd27e08d%3A0xb1c2554442d42e8d"
Then, assemble the URL for the API request. We’ll also set up headers for authentication and make the request:
url = f"https://api.hasdata.com/scrape/google-maps/reviews?{identifier}={identifier_value}"
headers = {
'Content-Type': 'application/json',
'x-api-key': API_KEY
}
response = requests.get(url, headers=headers)
Finally, we process the response. If the request is successful, we save the data to a CSV file. If it fails, we’ll print an error message so you can figure out what went wrong:
if response.status_code == 200:
data = response.json()
with open('reviews.csv', mode='w', newline='', encoding='utf-8') as file:
writer = csv.writer(file)
writer.writerow(['User Name', 'User Link', 'Rating', 'Date', 'Snippet', 'Review Link', 'Likes'])
for review in data.get('reviews', []):
writer.writerow([
review['user'].get('name', 'N/A'),
review['user'].get('link', 'N/A'),
review.get('rating', 'N/A'),
review.get('date', 'N/A'),
review.get('snippet', 'N/A'),
review.get('link', 'N/A'),
review.get('likes', 'N/A')
])
else:
print(f"Error. Status Code: {response.status_code}")
You can also change this step to customize the data you’re saving or add some preprocessing before writing it to the file. Here’s what the final dataset looks like:
In this example, we’re only fetching one page of reviews. If you want more, you’ll need to include the next page token in your API requests. This token is returned in the API response, so you can use it to get the next batch of reviews.
Scrape Reviews from Search Results
You can use the following example to scrape reviews from all the Google Maps places found on the map based on a specific query. If you’re looking for a ready-to-go solution, here’s the final Python code:
import requests
import csv
API_KEY = 'YOUR-API-KEY'
keyword = "Pizza"
output_file = "places_reviews.csv"
def get_places(keyword):
url = f"https://api.hasdata.com/scrape/google-maps/search?q={keyword}"
headers = {'Content-Type': 'application/json', 'x-api-key': API_KEY}
response = requests.get(url, headers=headers)
if response.status_code == 200:
return response.json().get('localResults', [])
else:
print(f"Error fetching places: {response.status_code}")
return []
def get_reviews(data_id):
url = f"https://api.hasdata.com/scrape/google-maps/reviews?dataId={data_id}"
headers = {'Content-Type': 'application/json', 'x-api-key': API_KEY}
response = requests.get(url, headers=headers)
if response.status_code == 200:
return response.json().get('reviews', [])
else:
print(f"Error fetching all the reviews for {data_id}: {response.status_code}")
return []
def collect_data(keyword, output_file):
places = get_places(keyword)
with open(output_file, mode='w', newline='', encoding='utf-8') as file:
writer = csv.writer(file)
writer.writerow([
'Place Name', 'Place ID', 'Total Reviews', 'Rating', 'User Name', 'User Link',
'User Rating', 'Review Date', 'Review Snippet', 'Review Likes'
])
for place in places:
if place['reviews'] > 0:
place_name = place.get('title', 'N/A')
place_id = place.get('placeId', 'N/A')
data_id = place.get('dataId', 'N/A')
total_reviews = place.get('reviews', 0)
rating = place.get('rating', 'N/A')
reviews = get_reviews(data_id)
for review in reviews:
writer.writerow([
place_name,
place_id,
total_reviews,
rating,
review['user'].get('name', 'N/A'),
review['user'].get('link', 'N/A'),
review.get('rating', 'N/A'),
review.get('date', 'N/A'),
review.get('snippet', 'N/A'),
review.get('likes', 'N/A')
])
if __name__ == "__main__":
collect_data(keyword, output_file)
print(f"Data collection completed. Results saved to {output_file}")
In this version, we’ve extracted the code for calling the Google Maps Reviews API from the previous example into a separate function, get_reviews(), and moved the logic for saving the data into collect_data(). The main change is that we added a call to the Google Maps API to get a list of places, along with their IDs and the number of reviews:
def get_places(keyword):
url = f"https://api.hasdata.com/scrape/google-maps/search?q={keyword}"
headers = {'Content-Type': 'application/json', 'x-api-key': API_KEY}
response = requests.get(url, headers=headers)
if response.status_code == 200:
return response.json().get('localResults', [])
else:
print(f"Error fetching places: {response.status_code}")
return []
We’ve added a filter in the collect_data() function to prevent empty results from places with no reviews. This ensures that we only scrape reviews for places with one or more reviews.
The result is a file that looks like this:
As you can see, this script can still be improved further to scrape even more reviews for each place.
Method 3: Scrape Google Maps Reviews Without Code
This method doesn’t require any programming skills; all you need to do is enter the data you’re interested in to collect reviews. To get started, head over to your account on our website, go to the “No-Code Scrapers” section, and find the Google Maps Reviews Scraper:
On the scraper page, specify the number of reviews you want to collect, provide the link to the Google Maps location, and, if you’d like, choose a sorting option:
Next, just run the scraper and wait for it to finish. Once the scraper finishes, you can download the data in one of the available formats: CSV, XLSX, or JSON. You’ll get all the details about the reviews like this:
[
{
"date": "2 weeks ago",
"isoDate": "2024-12-09T03:02:46.087Z",
"rating": 5,
"snippet": "Wow, what an incredible experience! The views are absolutely stunning – pictures don’t do it justice. There are plenty of spots to stop for photos while driving along the rim, the views are gorgeous from everywhere. We stayed in the village for one night and it was very convenient to be close to the most popular viewpoints. The visitor center has helpful info, and the staff is super friendly. We didn’t get a chance to hike but I would highly recommend it if you have more time. Definitely a must-see if you are driving through.",
"source": "Google",
"responseDate": "",
"responseIsoDate": "",
"responseSnippet": "",
"likes": 0,
"url": "https://www.google.com/maps/reviews/data=!4m8!14m7!1m6!2m5!1sChdDSUhNMG9nS0VJQ0FnSUN2dlBlWDNBRRAB!2m1!1s0x0:0xb1c2554442d42e8d!3m1!1s2@1:CIHM0ogKEICAgICvvPeX3AE%7CCgsI1rvZugYQwM7QKQ%7C?hl=en-US",
"userName": "Daria Kurovskaya",
"userProfileLink": "https://www.google.com/maps/contrib/111542442643050280292?hl=en-US",
"userPhotos": 81,
"userReviews": 62,
"userThumbnail": "https://lh3.googleusercontent.com/a-/ALV-UjVkjExshA-NJpU3ywgYK3vh7jqxGyqIpRKmADeQGnh5M9lxwdbj=s120-c-rp-mo-ba4-br100",
"isUserLocalGuide": true,
"placeUrl": "https://www.google.com/maps/place/Grand+Canyon/@36.099796,-112.1299942,14z/data=!3m1!4b1!4m5!3m4!1s0x80cc0654bd27e08d:0xb1c2554442d42e8d!8m2!3d36.0997631!4d-112.1124846",
"dataId": "0x80cc0654bd27e08d:0xb1c2554442d42e8d",
"images": "https://lh5.googleusercontent.com/p/AF1QipM2bJSxbQJyU-XLVXJWfSWanMSJXcM-V2UwPwOY=w150-h150-k-no-p, https://lh5.googleusercontent.com/p/AF1QipMFc1tlexXIM00JTqwbTrb_De2W70Zt78aBYBOb=w150-h150-k-no-p, https://lh5.googleusercontent.com/p/AF1QipN5EAw2tQs72IOSK3lAVV7pB9gMRStwhiQJoOpB=w150-h150-k-no-p, https://lh5.googleusercontent.com/p/AF1QipMR-oYTe4qj0nuYN8_9QawxqldIthbyIJCRgaq7=w150-h150-k-no-p, https://lh5.googleusercontent.com/p/AF1QipPR9QnJQsi_wCbTmL-DzDZfYGfqtY-XpJlYn3gS=w150-h150-k-no-p"
},
…
]
Overall, this is the easiest method for collecting reviews we’ve covered. It’s perfect for anyone, no matter what your level of technical experience is.
Conclusion
There you have it, we looked at the most common ways to scrape Google Reviews data ranging from the most complex approach to the easiest no-code reviews scraping method. If you are a true, hardcore developer seeking complete control over the process and are prepared to dedicate time not only to development but also to ongoing maintenance and updates, then creating your own scraper is the best option. If you’re up for some coding but don’t want to deal with proxies, constantly changing selectors, or just want to save time, the Google Maps Reviews API is the best option.
However, if you want an easy and quick way of seeing Google Reviews go with a no-code scraper. It will save you time and the potential headache of having troubleshooting your code. It also provides you with clean data free of empty results.
Might Be Interesting
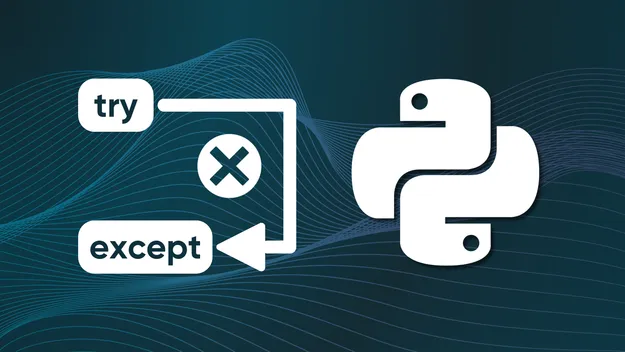
Feb 10, 2025
How To Retry Failed Python Requests
Learn how to implement retry mechanisms in Python Requests to handle timeout errors, HTTP status codes like 403, 429, 500, 502, 503, and 504, and avoid infinite loops with effective backoff strategies.
- Python
- Tools and Libraries
- Tutorials and guides
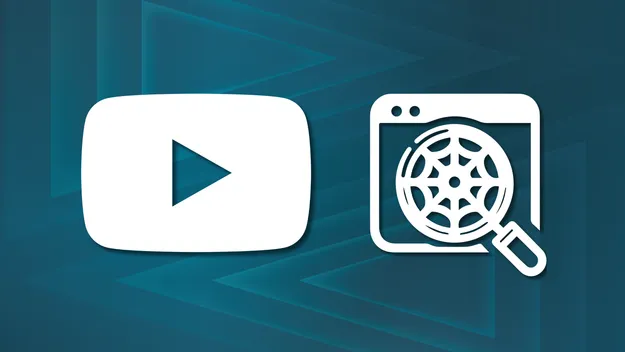
Oct 29, 2024
How to Scrape YouTube Data for Free: A Complete Guide
Learn effective methods for scraping YouTube data, including extracting video details, channel info, playlists, comments, and search results. Explore tools like YouTube Data API, yt-dlp, and Selenium for a step-by-step guide to accessing valuable YouTube insights.
- Python
- Tutorials and guides
- Tools and Libraries
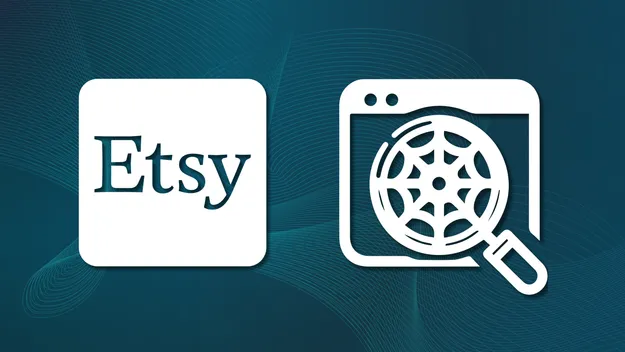
Oct 16, 2024
Scrape Etsy.com Product, Shop and Search Results Data
Learn how to scrape Etsy product, shop, and search results data with methods like Requests, BeautifulSoup, Selenium, and web scraping APIs. Explore strategies for data extraction and storage from Etsy's platform.
- E-commerce
- Tutorials and guides
- Python