Python and JavaScript are the most popular programming languages for web scraping. In this article, we'll delve into the strengths and weaknesses of both languages, providing their comparison. We'll explore specific use cases where JavaScript or Python might be better for your web scraping projects.
If you're interested in learning other programming languages for web scraping, we recommend reading our article on the best languages for web scraping. In that piece, we discuss the pros and cons of each language and provide basic examples of scrapers in action.
Get the power of web scraping without the hassle. The HasData web scraping API easily handles headless browsers and proxy rotation, so you can easily extract valuable…
Get fast, real-time access to structured Google search results with our SERP API. No blocks or CAPTCHAs - ever. Streamline your development process without worrying…
JavaScript for Web Scraping
JavaScript is the most widely used language in web development, with specific strengths and limitations for extracting data from websites. Its ability to interact easily with dynamic content gives it a strong advantage.
In this section, we'll look at popular JavaScript libraries used for web scraping, exploring their features and how they help with data extraction. We'll also discuss the benefits and challenges of using JavaScript.
After that, we'll compare JavaScript with Python, examining how well Python works for web scraping and how its approach differs from JavaScript.
Popular Libraries and Tools
Our previous article looked at the top 6 JavaScript and Node.js libraries for web scraping, including their advantages, disadvantages, and code examples. In this article, we’ll focus on the three most popular ones: Axios, Cheerio, and Puppeteer. The other libraries are less common and repeat what these three can do.
Axios and Cheerio are usually used together in web scraping. Axios is great for making requests to a website, and Cheerio helps you parse and work with the data you get back. They are easy to use and work well for straightforward scraping tasks.
Let’s use a demo website to demonstrate. We’ll create a simple script to pull out key product information from a page.
const axios = require('axios');
const cheerio = require('cheerio');
const url = 'https://demo.opencart.com/';
axios.get(url).then(response => {
const $ = cheerio.load(response.data);
const products = [];
$('.product-thumb').each((index, element) => {
const product = {
title: $(element).find('.description h4 a').text().trim(),
description: $(element).find('.description p').text().trim(),
price: $(element).find('.price .price-new').text().trim(),
tax: $(element).find('.price .price-tax').text().trim(),
image: $(element).find('.image img').attr('src'),
link: $(element).find('.description h4 a').attr('href'),
};
products.push(product);
});
console.log(products);
}).catch(error => {
console.error('Error:', error);
});
As you can see, these libraries allow you to extract data from relatively simple websites quickly. However, they can't handle dynamic content. For a deeper dive into these two libraries, check out our article on web scraping with Axios and Cheerio.
Another useful JavaScript library for scraping is Puppeteer. Unlike the others, Puppeteer is all-in-one and doesn't need extra tools. It lets you open a browser, visit pages, and simulate user actions.
Because Puppeteer can handle dynamic content loaded by JavaScript, so it's a great choice. Let's update the previous example to use Puppeteer:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://demo.opencart.com/', { waitUntil: 'networkidle2' });
const products = await page.evaluate(() => {
const productElements = document.querySelectorAll('.product-thumb');
const products = [];
productElements.forEach(element => {
const product = {
title: element.querySelector('.description h4 a').innerText.trim(),
description: element.querySelector('.description p').innerText.trim(),
price: element.querySelector('.price .price-new').innerText.trim(),
tax: element.querySelector('.price .price-tax').innerText.trim(),
image: element.querySelector('.image img').src,
link: element.querySelector('.description h4 a').href
};
products.push(product);
});
return products;
});
console.log(products);
await browser.close();
})();
By using this code, we can reliably extract data from web pages. It starts a web driver, visits the desired page, waits for it to fully load, and then efficiently collects the needed information. This method prevents errors caused by trying to scrape data before it's fully loaded.
Advantages of Using JavaScript
JavaScript is great for web scraping because it lets you collect data directly on the client side, meaning you can work directly within the browser. This is especially useful for dealing with dynamic web pages that change content frequently. With JavaScript, you can run scripts on the page you're scraping.
Node.js is another option. It lets you run JavaScript on the server side, which can be powerful for scraping. Furthermore, JavaScript's ability to handle multiple tasks simultaneously (asynchronous operations) makes it efficient for quickly collecting large amounts of data.
Effortlessly integrate web scraping into your Node.js projects with HasData's Node.js SDK, leveraging headless browsers, proxy rotation, and JavaScript rendering…
The Node.js Google Maps Scraping Library empowers developers to extract comprehensive location information from Google Maps effortlessly. This library streamlines…
Disadvantages of Using JavaScript
However, JavaScript has some downsides. It can be more complicated for beginners to learn than languages like Python. This complexity might make the development process harder.
Although there are many JavaScript libraries for scraping, tools for processing and analyzing data can be less extensive. Also, creating specialized tools, like those for handling PDFs, might need extra work and custom solutions.
Python for Web Scraping
Python has become a very popular option for web scraping in recent years. It's known for being easy to use and has many libraries and tools that help developers work on various data-related projects. Its clear and straightforward syntax has made it a favorite among the web scraping community.
Popular Libraries and Tools
Python has many libraries and tools for extracting, processing, and analyzing data. Let's compare this with the JavaScript libraries we’ve looked at before. The main Python tools for scraping data are Requests, BeautifulSoup, Selenium, and the Scrapy framework.
There are other Python libraries for scraping, but we'll focus on these popular ones. We’ve already covered examples of all these major Python scraping tools in other articles.
Requests and BeautifulSoup are especially easy for beginners and are commonly used. They simplify sending requests to websites and handle the HTML that comes back. They work similarly to JavaScript’s Axios and Cheerio, giving you effective tools for web scraping.
import requests
from bs4 import BeautifulSoup
url = 'https://demo.opencart.com/'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
products = []
for product in soup.find_all('div', class_='product-thumb'):
title = product.find('h4').text.strip()
description = product.find('p').text.strip()
price = product.find('span', class_='price-new').text.strip()
tax = product.find('span', class_='price-tax').text.strip()
image = product.find('img')['src']
link = product.find('h4').find('a')['href']
products.append({
'title': title,
'description': description,
'price': price,
'tax': tax,
'image': image,
'link': link
})
print(products)
Unlike JavaScript, this Python script will run synchronously by default. To make it asynchronous, you should use the aiohttp library instead of Requests. The aiohttp library works with asyncio to allow for asynchronous requests.
There are a few options for web drivers and browser automation in Python. The most popular ones are Pyppeteer, a wrapper around Puppeteer, and Selenium. Selenium is more widely used in Python, has a larger community, and better documentation. So, we'll use Selenium as an example.
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
from webdriver_manager.chrome import ChromeDriverManager
chrome_options = Options()
chrome_options.add_argument('--headless')
chrome_options.add_argument('--disable-gpu')
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()), options=chrome_options)
url = 'https://demo.opencart.com/'
driver.get(url)
products = []
product_elements = driver.find_elements(By.CLASS_NAME, 'product-thumb')
for product in product_elements:
title = product.find_element(By.CSS_SELECTOR, '.description h4 a').text.strip()
description = product.find_element(By.CSS_SELECTOR, '.description p').text.strip()
price = product.find_element(By.CSS_SELECTOR, '.price .price-new').text.strip()
tax = product.find_element(By.CSS_SELECTOR, '.price .price-tax').text.strip()
image = product.find_element(By.CSS_SELECTOR, '.image img').get_attribute('src')
link = product.find_element(By.CSS_SELECTOR, '.description h4 a').get_attribute('href')
products.append({
'title': title,
'description': description,
'price': price,
'tax': tax,
'image': image,
'link': link
})
driver.quit()
print(products)
To learn more about web scraping, check out our other article for a complete guide on using Selenium with Python.
Also, let’s consider the Scrapy framework. It’s great for building large-scale web scrapers and collecting big data. Scrapy is powerful and efficient, but setting it up can be tricky, so another article about scraping using Scrapy details it.
Although Python has more web scraping libraries than JavaScript, many of these libraries offer similar features.
Advantages of Using Python
Python has become extremely popular in recent years because it has many great features. It's easy to learn and use, making it a good choice for beginners and experienced programmers. Python has many libraries and tools that can help with many tasks.
One of Python's biggest strengths is its ability to work on data-based projects. Whether you're collecting, processing, or analyzing data, Python provides powerful tools to get the job done efficiently. This makes it a top choice for data-related projects.
Because so many people use Python, there is a large and active community of users. This community can be very helpful when working on projects like web scrapers or other tools. If you run into any problems, finding solutions or getting advice from other Python developers is easy.
The HasData Python SDK simplifies web scraping by handling complex tasks like browser rendering, proxy management, and CAPTCHA avoidance, allowing you to focus on…
The Google Maps API Python library offers developers an efficient means to harness detailed location data directly from Google Maps. This library simplifies the…
Disadvantages of Using Python
One drawback of using Python for data scraping is that it is often considered synchronous, which means it processes tasks one at a time. This can make it slower when you need to handle many requests simultaneously. On the other hand, JavaScript, particularly Node.js, is designed to handle asynchronous operations efficiently, making it better suited for scenarios involving multiple concurrent requests.
Additionally, Python generally has fewer built-in tools for interacting with dynamic content than JavaScript. JavaScript's capabilities, such as those provided by browser automation tools like Puppeteer, can be particularly useful for scraping data from websites that frequently update their content.
Despite these limitations, Python remains a popular and effective choice for web scraping. It boasts a range of powerful libraries, which simplify the process of extracting data from websites
Comparison of JavaScript and Python for Web Scraping
Before we dive into a detailed comparison of JavaScript and Python, let's start with a simple table to highlight the main features of each programming language:
Aspect |
JavaScript (Node.js) |
Python |
---|---|---|
Main Libraries |
- axios |
- requests |
- node-fetch |
- aiohttp |
|
- puppeteer |
- BeautifulSoup |
|
- cheerio |
- Scrapy |
|
- request (deprecated) |
- Selenium |
|
Concurrency |
- Native support with async/await |
- Native support with asyncio |
- Promise |
- aiohttp for asynchronous requests |
|
- Efficient with puppeteer for headless browsing |
- concurrent.futures for multi-threading |
|
Ease of Use |
- Modern async syntax is easy to use for concurrency |
- BeautifulSoup is user-friendly for HTML parsing |
- cheerio for lightweight HTML parsing |
- Scrapy provides a full-featured scraping framework |
|
Performance |
- Generally fast with puppeteer and native async support |
- Asynchronous support with aiohttp is efficient |
- Suitable for handling multiple concurrent requests efficiently |
- Scrapy is optimized for large-scale scraping |
|
Handling JavaScript |
- Excellent support with puppeteer and playwright |
- Requires Selenium or headless browsers like Splash for JavaScript-heavy sites |
- Can handle dynamic content and interactive elements |
||
Community and Ecosystem |
- Large community with many modern tools |
- Rich ecosystem with a variety of scraping libraries and frameworks |
- Extensive libraries for web scraping and automation |
- Strong community support |
|
Learning Curve |
- May require learning asynchronous programming patterns |
- Generally straightforward for beginners |
- Libraries like puppeteer can have a steeper learning curve |
- Scrapy can be complex for large-scale projects |
|
Error Handling |
- Promises and async/await provide robust error handling mechanisms |
- Exceptions and async error handling with aiohttp and requests are well-supported |
Support for Headless Browsing |
- Excellent support with puppeteer, playwright |
- Selenium or external tools required for headless browsing |
As shown in the table, it's hard to say which programming language is the best for data scraping. Based on our experience, the most effective language for scraping data is usually the one you already know well. If you’re comfortable with a language, you’ll code more efficiently, fix problems faster, and get better results overall.
Ease of Use
Creating and using scripts can be easier or harder, depending on what programming languages you already know. For example, if you're familiar with JavaScript from working on websites, you'll find it straightforward to create a web scraper using JavaScript. Our detailed guide walks you through the steps of making a scraper with JavaScript or Node.js and how to run it.
Learning JavaScript can be very helpful for web scraping, even for Python developers. Sometimes, you might need to include JavaScript snippets in your main Python script to handle tasks like scrolling through pages or clicking buttons.
On the other hand, if you're new to programming and want to start web scraping, Python is a great choice. It's generally easier to learn compared to other programming languages. Our tutorial introduces you to Python and web scraping, including practical examples using popular libraries.
Popularity
The popularity of a programming language can vary depending on how you measure it. In this section, we'll look at how popular JavaScript and Python are based on some objective data sources.
One useful tool for this analysis is Google Trends. Google Trends shows how often people search for terms like "JavaScript" and "Python" over time. You can adjust the search to focus on specific regions and periods to get a clearer picture of how interest in these languages changes.
This way, we can see which language is being searched for more frequently and understand trends in their popularity.
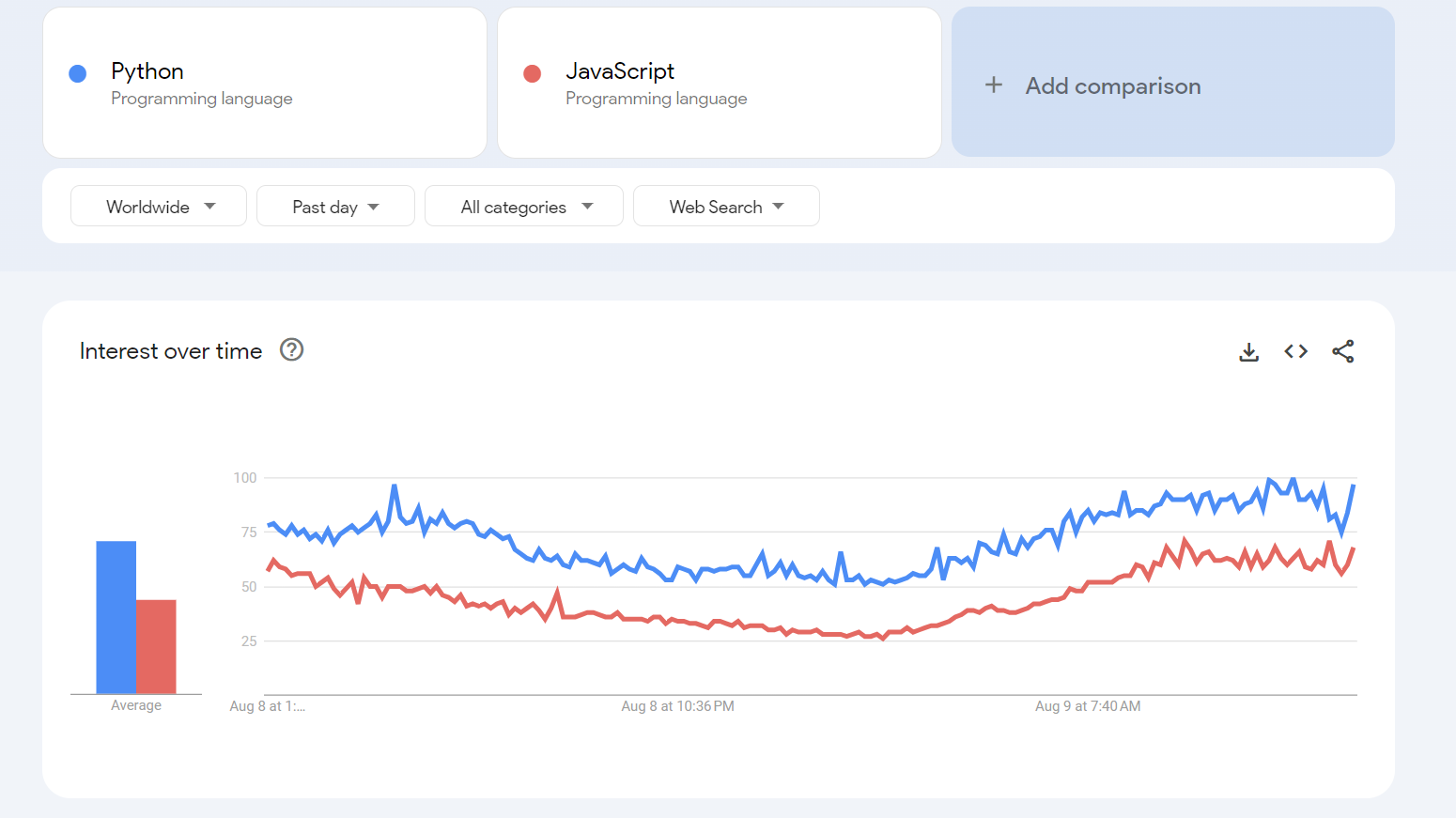
As shown in the graph, the data from the platform indicates that Python is more popular. However, as mentioned earlier, relying on data from a single source may not provide a complete picture. Therefore, let’s move on to the next platform for a broader perspective.
The next service commonly used to evaluate the popularity of programming languages is Tiobe. Tiobe's rankings are based on the number of search queries related to various programming languages across major search engines like Google, Bing, Yahoo, Wikipedia, Amazon, and others. This method helps to provide a more comprehensive view of how frequently programming languages are searched and discussed online, offering a better understanding of their overall popularity.
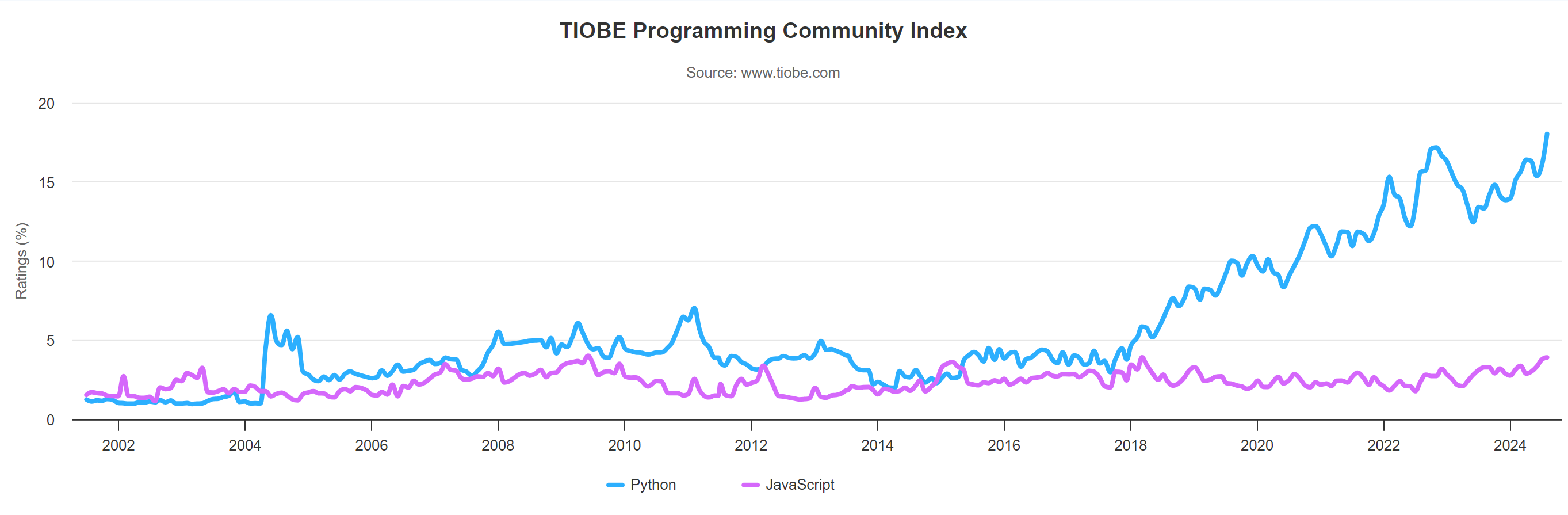
This index is updated monthly and shows programming language trends over the past 20 years. According to the Tiobe Index for August 2024, Python is currently the most popular programming language, while JavaScript is in sixth place.
Finally, let’s look at GitHub Octoverse, which I find to be a highly reliable and detailed source. GitHub Octoverse provides insights into the number of projects on GitHub, the world’s largest platform for hosting code. It also includes information about which programming languages are most commonly used in active projects.
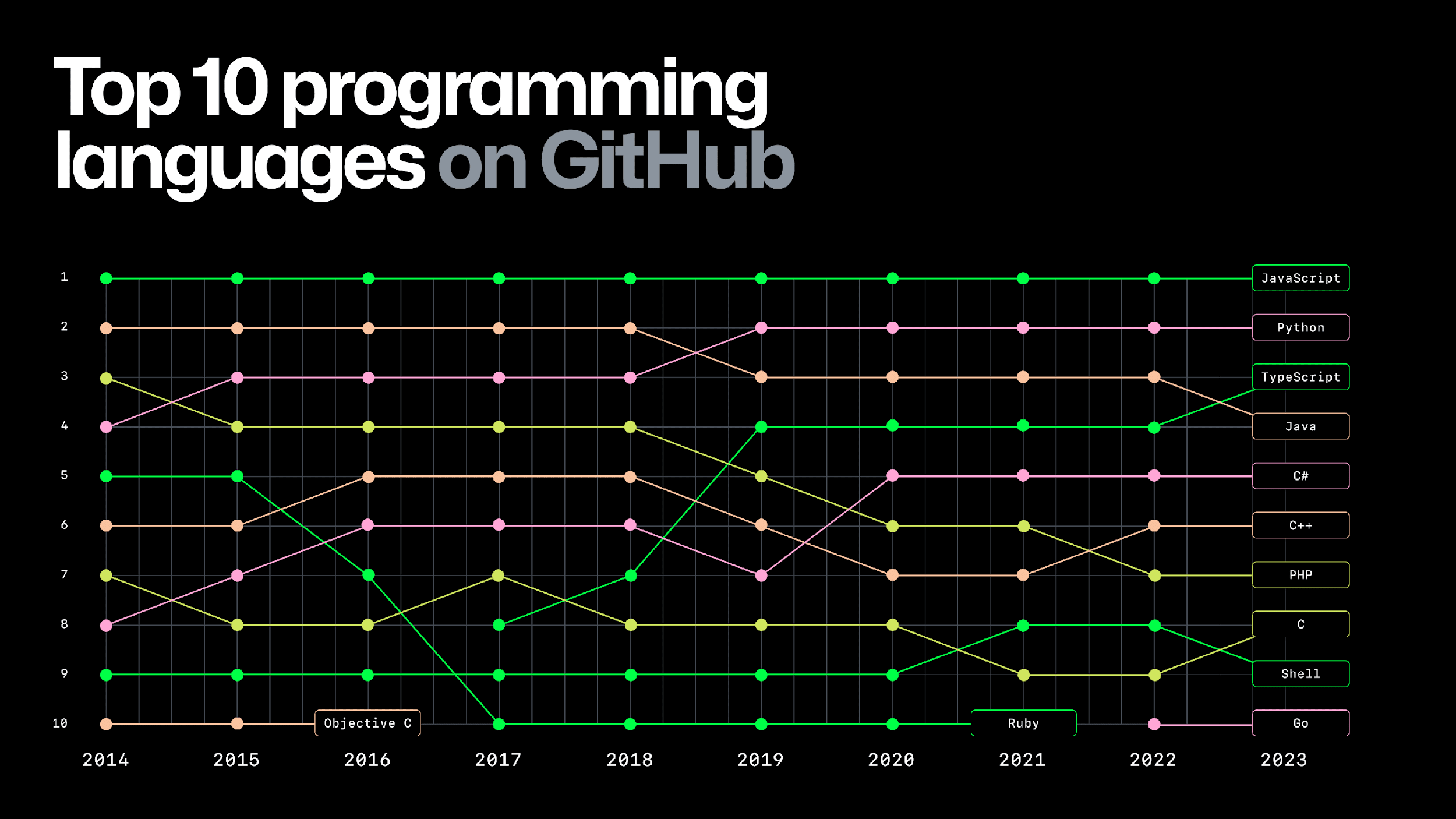
From this data, JavaScript is more popular on GitHub, as it is used in many projects. JavaScript has been leading in this respect since 2014. Therefore, while Python is very popular, it's still too early to call it the most popular programming language overall.
Performance
Evaluating the performance and efficiency of scripts written in different programming languages can be subjective. To get a clearer picture, let’s write two asynchronous scripts: JavaScript and Python. Both scripts will repeatedly fetch a demo website (10 times), measure how long each request takes, and then calculate the average and total time spent.
Before we dive into the code examples, it's important to understand why comparing different languages this way is useful. Running identical scripts on the same machine, using similar tools, and under the same conditions allows for a fair assessment of language performance in specific tasks:
- Web Scraping and Load Testing. We can see which language handles many requests better by running the same script in both JavaScript and Python. This is useful for tasks like web scraping or testing a website's performance under heavy load.
- Asynchronous and Parallel Programming. Different languages handle asynchronous tasks differently. Comparing them helps us understand how well each language manages tasks that run simultaneously and their impact on performance.
- Development, Maintenance, and Scalability. Beyond just speed, we also want to know how easy it is to write, maintain, and scale scripts in each language. Some languages might be more user-friendly or better suited for scaling up projects.
- Learning and Understanding Language Differences. We can understand each language’s unique features, strengths, and weaknesses by comparing practical examples. This helps us see how different languages tackle similar problems.
Testing scripts under controlled conditions helps us understand which language might best fit our needs. It also aids in making better decisions about development and optimization.
In our case, these tests can help you determine which language you find more comfortable to work with. Let's refine the JavaScript script we used earlier:
const axios = require('axios');
const { performance } = require('perf_hooks');
const url = 'https://demo.opencart.com';
async function fetch() {
const startTime = performance.now();
await axios.get(url);
const endTime = performance.now();
return endTime - startTime;
}
async function runScraper() {
const requestPromises = Array.from({ length: 10 }, () => fetch());
const times = await Promise.all(requestPromises);
return times;
}
async function main() {
const startTime = performance.now();
const requestTimes = await runScraper();
const totalExecutionTime = performance.now() - startTime;
const sumOfRequestTimes = requestTimes.reduce((a, b) => a + b, 0);
const averageRequestTime = sumOfRequestTimes / requestTimes.length;
console.log(`Total Execution Time: ${totalExecutionTime / 1000} seconds`);
console.log(`Average Request Time: ${averageRequestTime / 1000} seconds`);
}
main().catch(console.error);
Now, let's adapt the Python script we discussed earlier. We'll need to replace the requests library with aiohttp and asyncio, so our program can run asynchronously. In terms of functionality, we'll replicate the JavaScript script:
import aiohttp
import asyncio
import time
url = 'https://demo.opencart.com'
async def fetch(session, url):
start_time = time.time()
async with session.get(url) as response:
await response.text()
end_time = time.time()
return end_time - start_time
async def run_scraper():
async with aiohttp.ClientSession() as session:
tasks = [fetch(session, url) for _ in range(5)]
times = await asyncio.gather(*tasks)
return times
async def main():
start_time = time.time()
request_times = await run_scraper()
total_execution_time = time.time() - start_time
average_request_time = sum(request_times) / len(request_times)
print(f"Total Execution Time: {total_execution_time} seconds")
print(f"Average Request Time: {average_request_time} seconds")
asyncio.run(main())
Upon execution, we will obtain the following results:
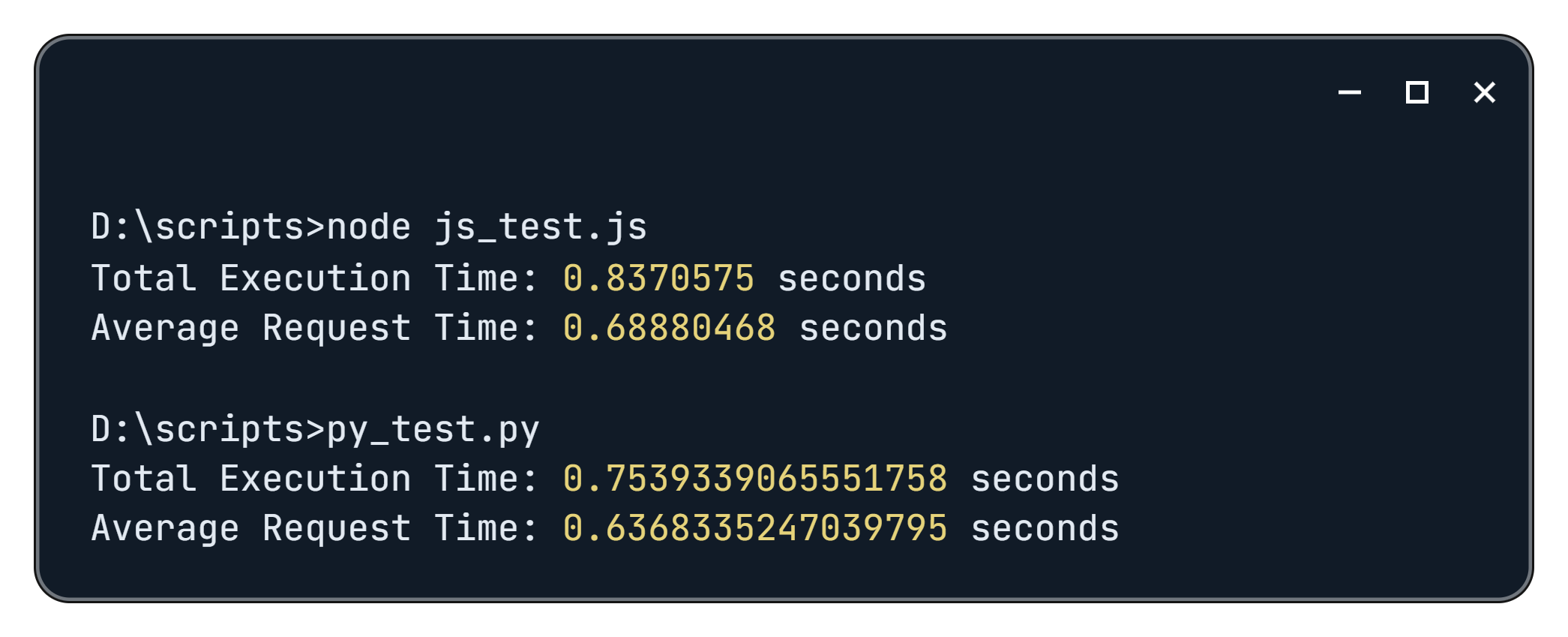
Let's not make any conclusions based on just one test run. We'll run the script 30 times in a loop to get more reliable results. This way, we'll collect enough data to make a better analysis.
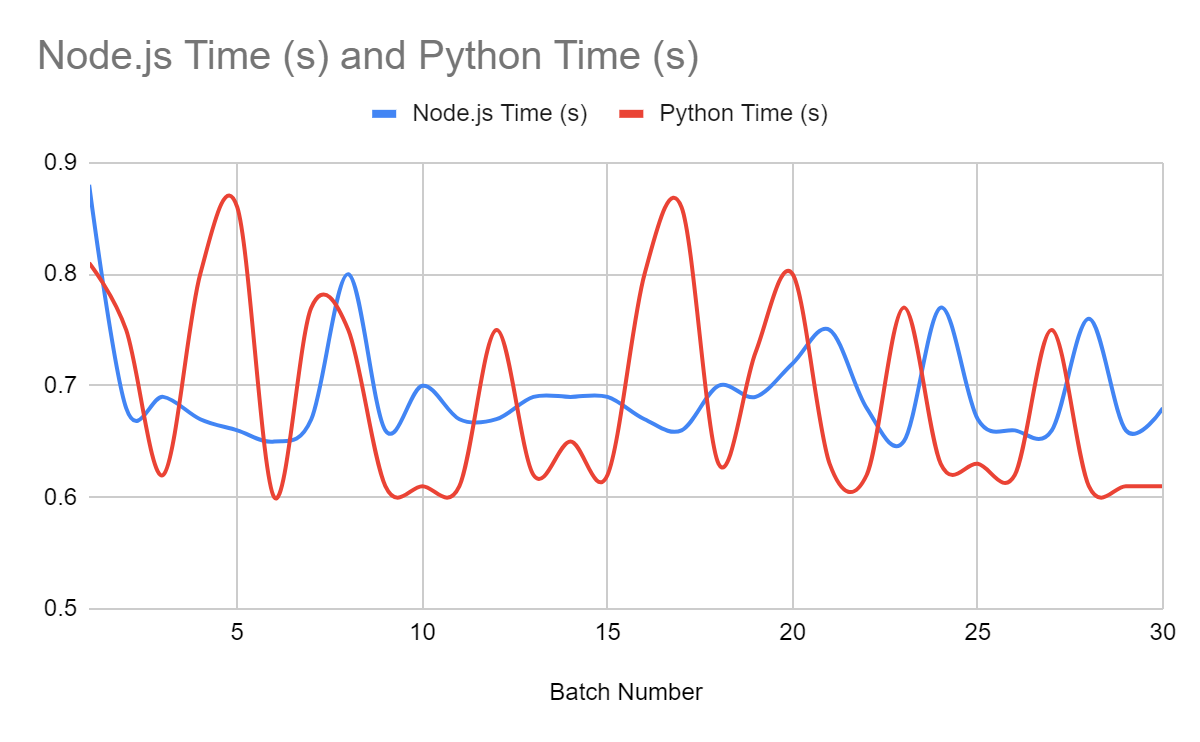
The graph shows that Python and JavaScript produce similar results for web scraping. This means that when creating a web scraper, the choice of programming language might not be very important. Both languages perform equally well in this task.
Conclusion and Takeaways
When choosing a programming language for web scraping, your decision largely depends on your familiarity with the language and project requirements. If you're skilled in Python, you might find writing a more efficient scraper easier than JavaScript, even though JavaScript has its advantages. If you're new to programming, it's important to consider the strengths and weaknesses of each language to find the best fit for your project.
When to Choose JavaScript
JavaScript is the best choice if:
- You are already familiar with JavaScript.
- You need to integrate the scraper into a JavaScript-based project.
- Your project involves elements that require JavaScript to function properly.
For instance, if you're building a browser extension that needs to collect data from web pages, JavaScript is a suitable choice because it runs directly in the browser environment. Similarly, if you're developing a tool for Google Sheets, which uses a simplified version of JavaScript called Google Apps Script to interact with and manipulate spreadsheet data.
When to Choose Python
Python is the best choice if:
- You are already familiar with Python.
- It is your first programming language.
- You want to do more than just collect data, you also plan to analyze and process it within the same script.
Python excels in projects that involve data analysis and machine learning, making it ideal for tasks that require handling and interpreting large datasets. Its extensive libraries and tools make it a powerful option for scraping and analyzing data efficiently.